index.jsp
<%@page import="com.demo.MessageList"%>
<%@ page language="java" import="java.util.*" pageEncoding="utf-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme() + "://"
+ request.getServerName() + ":" + request.getServerPort()
+ path + "/";
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base href="<%=basePath%>">
<title>留言本 - 无数据库版</title>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<style type="text/css">
<!--
textarea {
FONT: 12px Tahoma;
COLOR: #333333;
margin-top: 3px;
margin-bottom: 3px;
}
.input1 {
width: 300px;
height: 25px;
margin-top: 3px;
margin-bottom: 3px;
}
.input2 {
width: 150px;
height: 25px;
margin-top: 3px;
margin-bottom: 3px;
}
.submit1 {
width: 50px;
border: #CCCCCC solid 1px;
height: 30px;
background: #FFFFFF
}
#form1 tr th {
text-align: right;
}
input {
text-align: left;
}
body {
font-family: "微软雅黑", Verdana, sans-serif, "宋体";
color: #300;
margin-right: auto;
margin-left: auto;
text-align: center;
border: 1px solid #333;
}
.mybutton {
width: 70px;
height: 25px;
margin-top: 5px;
margin-bottom: 5px;
text-align: center;
}
h1 {
color: #333;
font-weight: bold;
font-size: 24px;
margin-bottom: 25px;
}
#message div .name {
float: right;
margin-right: 10px;
width: 100px;
}
#message .row {
width: 500px;
margin-right: auto;
margin-left: auto;
text-align: left;
font-size: 10px;
font-family: "微软雅黑", Verdana, sans-serif, "宋体";
border-left-width: 5px;
border-left-style: solid;
border-left-color: #999;
margin-bottom: 10px;
background-color: #f5f5f5;
}
#message .row .time {
float: right;
}
#message .row .msg {
text-indent: 2em;
font-size: 12px;
margin-top: 2px;
border-bottom-width: 1px;
border-bottom-style: dashed;
border-bottom-color: #FFF;
font-family: "微软雅黑", Verdana, sans-serif, "宋体";
font-weight: bold;
}
#message {
margin-bottom: 50px;
}
-->
</style>
<script>
function addCheck() {
var name = document.getElementById("name").value;
var msg = document.getElementById("msg").value;
if (name == "") {
alert("姓名不能为空!")
document.getElementById("name").focus();
return false;
}
if (msg == "") {
alert("留言不能为空!")
document.getElementById("title").focus();
return false;
}
}
</script>
</head>
<body>
<h1>
留言本(无数据库版)
</h1>
<div id="message">
<%
//输出所有留言
MessageList messageList = (MessageList) application
.getAttribute("MessageList");
if (messageList == null) {
out.println("留言本是空的!");
} else {
Iterator iterator = messageList.get().iterator();
while (iterator.hasNext()) {
String[] row = (String[]) iterator.next();
%>
<div class="row">
<div class="msg">
<%=row[1]%></div>
<div class="time">
<%=row[2]%>
</div>
<div class="name">
姓名:<%=row[0]%>
</div>
</div>
<%
}
}
%>
</div>
<table width="500" align="center" cellpadding="2" cellspacing="0">
<form name="form1" id="form1"
action="<%=basePath%>servlet/AddMessage" method="post"
onSubmit="javascript: return addCheck()">
<tr>
<th width="70" align="right">
姓名:
</th>
<td width="280" align="left">
<input name="name" type="text" class="input2" id="name">
</td>
</tr>
<tr>
<th>
<span class="STYLE1">留言</span>:
</th>
<td>
<textarea name="msg" id="content" cols="60" rows="5"></textarea>
</td>
</tr>
<tr>
<td colspan="2" align="center">
<input type="submit" name="submit" id="submit" value="提交留言"
class="mybutton" onsubmit="javascript:return addCheck()">
<input type="reset" name="reset" id="reset" value="重新填写"
class="mybutton">
</td>
</tr>
</form>
</table>
</body>
</html>
AddMessage.java
package com.demo;
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.Date;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
/**
* 添加留言
*
* @author Feng
*
*/
public class AddMessage extends HttpServlet {
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
}
public void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
request.setCharacterEncoding("utf-8");
ServletContext servletContext = request.getSession()
.getServletContext();
MessageList messageList = (MessageList) servletContext
.getAttribute("MessageList");
if (messageList == null) {
messageList = new MessageList();
}
SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
String currentTime = formatter.format(new Date());
String name = request.getParameter("name");
String msg = request.getParameter("msg");
String time = currentTime.toString();
messageList.add(name, msg, time);
servletContext.setAttribute("MessageList", messageList);
response.sendRedirect(request.getContextPath() + "/index.jsp");
}
}
MessageList.java
package com.demo;
import java.lang.reflect.Array;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import com.sun.org.apache.regexp.internal.recompile;
/**
* 留言列表
*
* @author Feng
*
*/
public class MessageList {
private List<String[]> messageList = new ArrayList<String[]>();
/**
* 添加留言
*
* @param name
* @param msg
* @param time
*/
public void add(String name, String msg, String time) {
String[] message = new String[3];
message[0] = name;
message[1] = msg;
message[2] = time;
this.messageList.add(message);
}
/**
* 获取全部留言
*
* @return
*/
public List<String[]> get() {
return this.messageList;
}
}
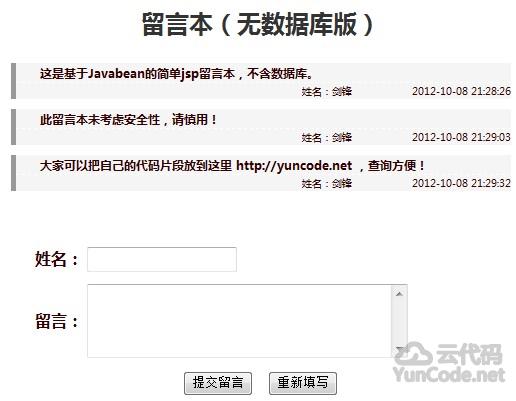
高级设计师
by: 由月君 发表于:2012-10-08 21:46:18 顶(1) | 踩(1) 回复
这个东西不错啊!
回复评论